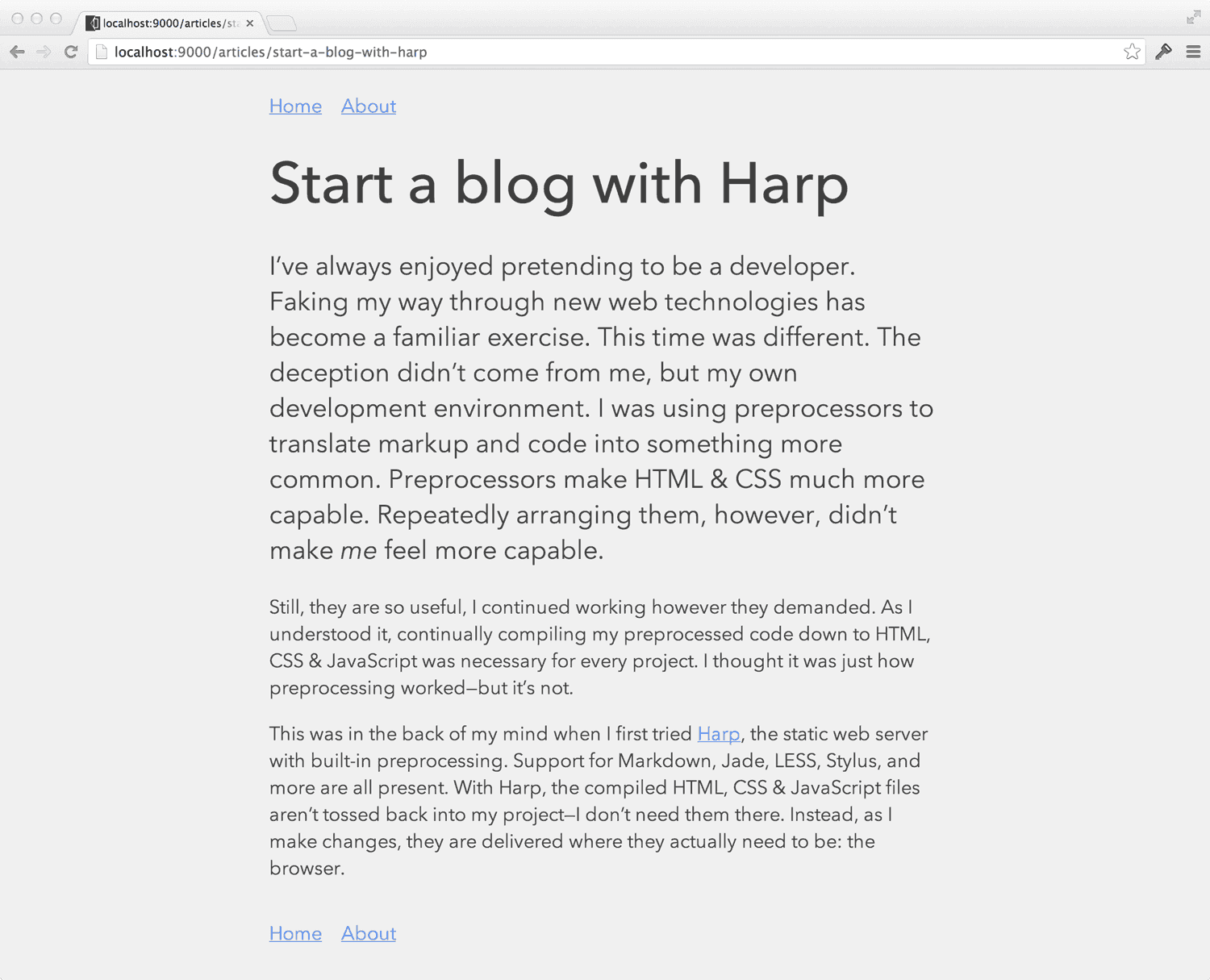
Start a blog with Harp
I’ve always enjoyed pretending to be a developer. Faking my way through new web technologies has become a familiar exercise. But this time was different: the deception didn’t come from me, but my own development environment.
I was using preprocessors to translate markup and code into something more common: HTML & CSS. Preprocessors make these front-end languages much more capable. Repeatedly configuring them, however, didn’t make me feel more capable.
Still, they are so useful, I continued working however they demanded. As I understood it, continually compiling my preprocessed code down to HTML, CSS & JavaScript was necessary for every project. I thought it was just how preprocessing worked.
This was in the back of my mind when I first tried Harp, the static web server with built-in preprocessing. Support for Markdown, Jade, LESS, Stylus, and more are all present. With Harp, the compiled HTML, CSS & JavaScript files aren’t tossed back into my project—I don’t need them there. Instead, as I make changes, they are delivered to where they actually need to be: the browser.
Since then, I’ve been incredibly lucky to get to work on Harp, in addition to making real projects with it. It’s removed my inconveniences preceding preprocessing. @sintaxi recently introduced Harp and showed it does a lot more.
Harp is open source, which helps makes it easy to start building something right away. Let’s make a simple blog—I am one of those people that makes more blogs than posts, after all.
Install Harp
First, you’ll need to install Node.js. Then, open the command prompt. If you’re not acquainted, that’s okay: it’s only needed sparingly. On OS X, you can use the Terminal, located in Applications → Utilities → Terminal. On Windows, Node.js will have come with the Node.js Command Prompt.
To install Harp, type in:
sudo npm install harp -g
You can leave off sudo
if you’re on Windows. Harp will now be installed globally.
Use cd
to navigate to the Documents folder (or wherever else you’d like), and initialise a new Harp app called my-harp-blog
:
cd Documents
harp init my-harp-blog
This will create a folder called my-harp-blog
with the default app inside, which looks like this:
▾ /
▪ _layout.jade
▪ 404.jade
▪ index.jade
▪ main.less
Live in the Browser
Now, you’ll want to start the web server and actually see the Harp app:
harp server my-harp-blog
Harp is now serving my-harp-blog
locally, so you can visit your app at localhost:9000.
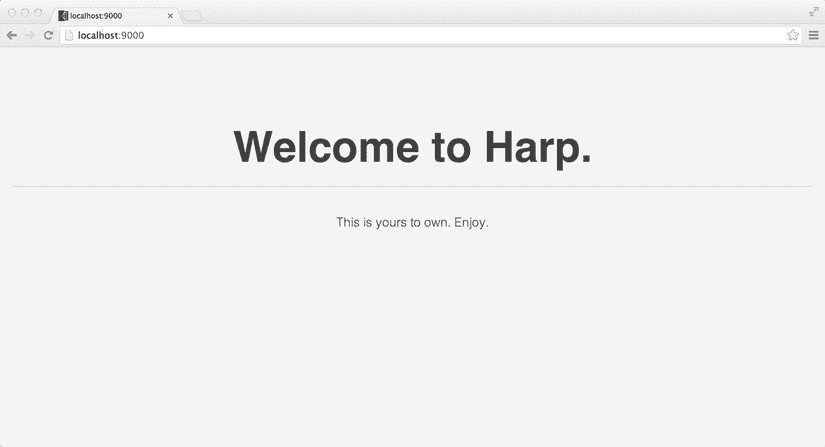
Start with Markdown
The default app contains as little as it can. This means you’ll be able to build your blog upon it without any trouble.
Adding a Markdown file is adding a new page. Create about.md
in the root directory, and add a little about yourself.
# About Kenneth
Hi, I’m Kenneth. Sometimes I write about building things with [Harp](http://harpjs.com).
Saving the file and visiting localhost:9000/about shows your about page. There was no need to stop and restart Harp or configure anything.
Make a change to the about.md
file—my content could be more helpful—save, and refresh the browser. Again, your changes are already there.
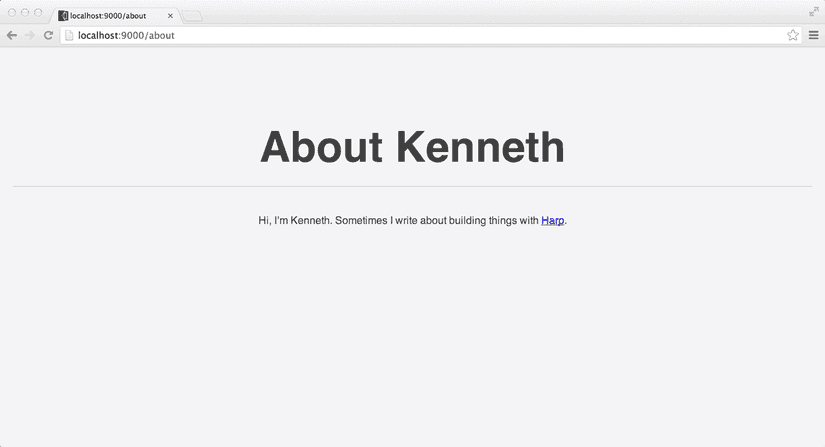
If you’ve worked with a static site generator, you may have had to start and stop its included file watcher. With Harp, this is unnecessary; as long as the server is running, changes are delivered to the browser. And, this process is very fast: files are only prepared as needed, so the entire application doesn’t need to be rebuilt when a single file is changed.
You may have noticed Harp didn’t just render the about page as plain HTML. Instead, it matched the index page, because it passed through a layout file first.
Layouts
Layouts are used for establishing repeating structures, whether it’s a header and footer or something more complicated. The _layout
file contains this markup. By convention, Harp doesn’t serve files or folders with an underscore preceding their name. Essentially, this _layout.jade
becomes a wrapper for the files that are served.
The content from your about.md
, 404.jade
and index.jade
are all brought into the layout wherever you use the variable yield
:
doctype
html
head
link(rel="stylesheet", href="/main.css")
body
!= yield
Jade’s clarity is compelling. I’d recommend giving it a chance even if it seems unfamiliar at first. Harp also supports EJS if you’d rather stick with HTML:
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="main.css" />
</head>
<body>
<%- yield %>
</body>
</html>
In Jade, the exclamation mark is preventing yield
from being escaped. The same is true of the hyphen in EJS.
With either, you’ll notice main.css
is referenced, even though that file isn’t actually in the folder; main.less
is. Because Harp has preprocessing built in, there’s no need to have the compiled files around, cluttering up your app. Update and save the LESS file, and your changes are already in the browser. I added a little to the default stylesheet. If you’d like to use the same one, it’s located here.
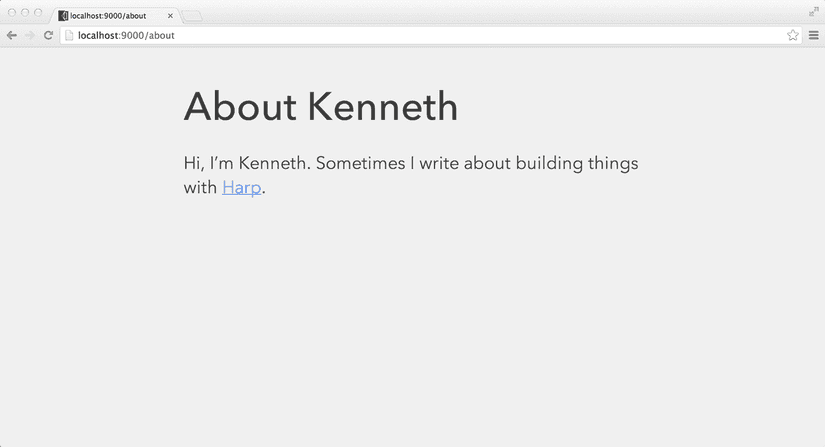
Adding Articles
You might want your blog to actually have some articles. Go ahead and write a few posts. I can wait.
Now, I would like to access my first article at localhost:9000/articles/another-article
, so I’ll create a folder called articles
. Folders are for people and so are URLs; make the directory structure mirror the URL you want.
▾ /
▪ _layout.jade
▪ 404.jade
▪ index.jade
▪ main.less
▾ articles/
▪ _data.json
▪ a-simple-article.md
▪ another-article.md
There is nothing unexpected going on inside the articles I added, they are just Markdown. Information about the articles, beyond the articles themselves, is stored inside the _data.json
file.
Flexible Metadata
Keeping metadata inside _data.json
, rather than inside each individual .md
file, works well for a number of reasons:
- A file can have any amount of metadata, and it still won’t interfere with your writing.
- Metadata for posts, images, videos or anything else can be added in the same way.
- Different files can still easily make use of this
_data.json
. - The order of information can come from the
_data.json
, rather than requiring anything of the file name
By adding the title, date, or any other information in a _data.json
file inside the article itself, you can get the most out of using Markdown and just worry about writing.
For this blog, I decided to just add the title in my _data.json
file for now:
{
"a-good-article": {
"title": "A Good Article"
},
"a-complicated-article": {
"title": "Another Article"
}
}
Using Jade, it’s possible to iterate over this metadata, listing out all the articles. I’ve added the following to index.jade
.
ul
each article, slug in public.articles._data
li
a(href="/articles/#{ slug }") #{ article.title }
As you’d expect, there’s a list of articles on the home page now.
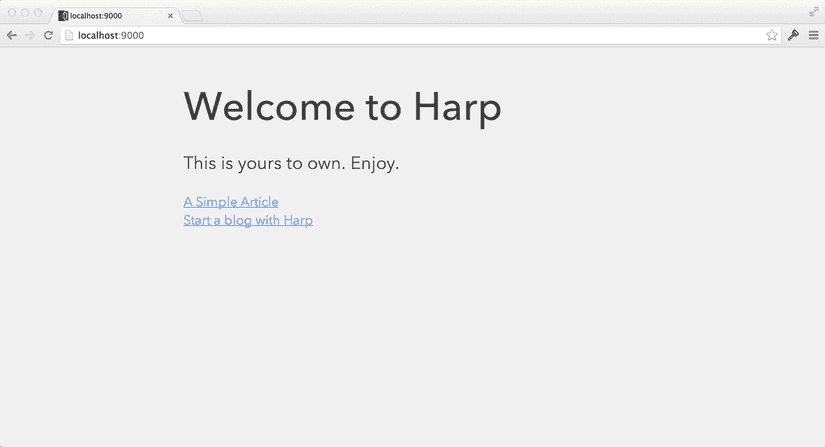
This blog is still missing a way to navigate. It would be nice to have the nav in the footer as well as the header of our layout, so it’s present when someone reaches the bottom of an article. Harp’s partial
function makes this possible without writing the same markup multiple times.
Partials
partial()
brings one file into another. You could use any text-based file in your application as a partial. Usually you’ll want to bring in snippets of code rather than entire pages, but you can use partial()
for either.
As with the _layout
file, adding an underscore to the beginning of a file’s name prevents it from generating its own page, which is great for snippets of code. This convention is true for folders, too: name a folder with an underscore at the beginning, and nothing inside will be served directly. Folders with a leading underscore are a great place to store partials. I created a new folder called _shared
for this purpose.
Inside _shared/
is a new file named nav.jade
.
nav
a(href="/") Home
a(href="/about") About
Now, in _layout.jade
, I’m using this file with partial()
:
doctype
html
head
link(rel="stylesheet", href="/main.css")
body
!= partial("_shared/nav")
!= yield
footer
!= partial("_shared/nav")
Now, the nav can go at the top and bottom of the blog posts without the markup needing to be duplicated. This is a simple example, but you can probably imagine how useful partials are once parts of your blog can be reused anywhere.
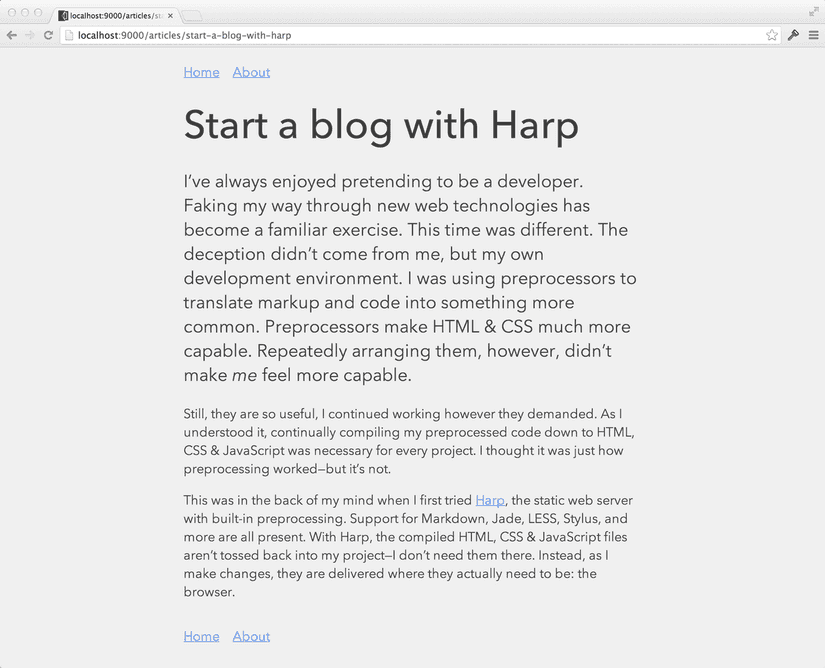
Getting it out there
This blog is looking more realised, certainly enough to put this first version online. A Harp app can be flattened into HTML, CSS & JavaScript, and published wherever you’d like.
harp compile
This command generates a www
folder filled with your blog’s HTML, CSS & JavaScript. It works great wherever you release your projects, including Amazon S3, GitHub Pages, Heroku, or even Apache Cordova/PhoneGap.
We’re also working on the Harp Platform, which lets you skip the compile step entirely and publish your app via your Dropbox.
Surge.sh is a more recent iteration on the aforementioned idea, and supports publishing sites built with Harp or any other kind of static site generator. You can read more about my work on Surge, or read “Make something of your ridiculous domains” and get your Harp project online.
I’ve found working with pre-processors like LESS, Markdown, and Jade less convoluted now that I’m building sites with Harp. Everything’s as it should be: the only awkward part of my development environment is me.